Variables and comments in Vagrantfile
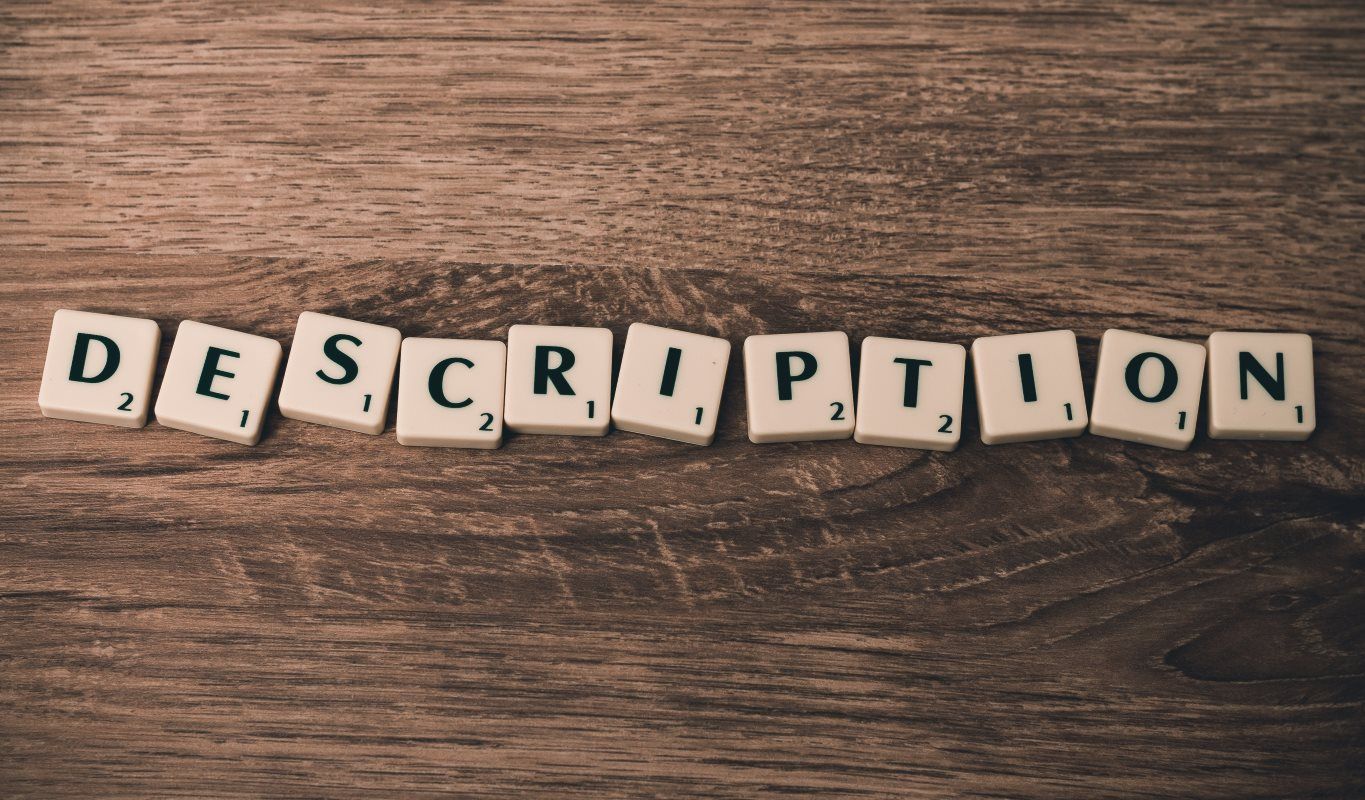
There might come a time when you need to improve a Vagrantfile beyond the basic elements. Here are a couple of tricks that might make some things easier.
Variables
There is a way to define variables in Vagrantfile for easier management of constants and settings, especially if having more than 1 box configured in Vagrantfile. A sample Vagrantfile with variables could look like this:
### configuration parameters ###
# which host port to forward box SSH port to
LOCAL_SSH_PORT = "2222"
# Vagrant base box to use
BOX_BASE = "puppetlabs/centos-6.6-64-nocm"
# amount of RAM for Vagrant box
BOX_RAM_MB = "2048"
# number of CPUs for Vagrant box
BOX_CPU_COUNT = "1"
### /configuration parameters ###
Vagrant.require_version ">= 1.8.0"
Vagrant.configure("2") do |config|
config.ssh.username = "vagrant"
config.ssh.insert_key = false
config.ssh.host = "127.0.0.1"
config.ssh.guest_port = 22
config.vm.box_check_update = false
config.vm.define "testbox" do |testbox|
testbox.vm.box = BOX_BASE
testbox.vm.hostname = "testbox"
testbox.vm.network :forwarded_port, guest: 22, host: LOCAL_SSH_PORT, id: "ssh", auto_correct: true
testbox.vm.provider :virtualbox do |virtualbox|
virtualbox.customize ["modifyvm", :id, "--memory", BOX_RAM_MB]
virtualbox.customize ["modifyvm", :id, "--cpus", BOX_CPU_COUNT]
end
end
end
Comments
Sometimes it is needed to comment out a bigger piece of Vagrantfile code. Adding hashtags to every line is an option, but another way is to comment out a block at once. The way to do that is prepending =begin
to the start and =end
at the of the block. The line with these delimiters must not begin with a whitespace. It is a bit more difficult to set up, but seems more convenient to remove when needed:
=begin
some.stuff "to" do |here|
do.some.other = stuff
done
=end
The code from the previous example might look something like this:
=begin
config.vm.define "testbox" do |testbox|
testbox.vm.box = BOX_BASE
testbox.vm.hostname = "testbox"
testbox.vm.network :forwarded_port, guest: 22, host: LOCAL_SSH_PORT, id: "ssh", auto_correct: true
testbox.vm.provider :virtualbox do |virtualbox|
virtualbox.customize ["modifyvm", :id, "--memory", BOX_RAM_MB]
virtualbox.customize ["modifyvm", :id, "--cpus", BOX_CPU_COUNT]
end
end
=end